All Packages Class Hierarchy This Package Previous Next Index
Class kraft.monitor.display.MonitorGUI
java.lang.Object
|
+----java.awt.Component
|
+----java.awt.Container
|
+----java.awt.Panel
|
+----kraft.monitor.display.MonitorGUI
-
public class MonitorGUI
-
extends Panel
-
implements ActionListener, AdjustmentListener, ItemListener, MonitorServer
This is the backbone to the Monitor program. Users must provide an object
that calls the monitoring methods made available via the MonitorServer
interface. This is especially important if the methods are intended to
be called remotely, as this class does not perform the necessary binding
to a Naming service or exporting as a remote object (as the object would
be unnecessarily large, a smaller object should be used for this purpose)
See LinjaWrapper for an example of a calling object that does more -- it
is not intended to be remote to this GUI as it hooks into a linda server,
and receives duplicate messages, which it then converts into a format usable
by this GUI (Message/Monitorable interfaces). Provides a graphical interface
which forms a useful visualisation tool to visualise the interactions in
a co-operating agent system.
Also deals with providing a Graphical User Interface, with buttons and
a slider to allow stepwise movement (backwards and forwards) through the
visualisation
-
Author:
-
Ted Francis
-
See Also:
-
MonitorCanvas,
LinjaAdapter

-
BOTTOM
-
Constant used in output()
-
canvas
-
FAST
-
Constants for use with the setDelay method.
-
MEDIUM
-
Constants for use with the setDelay method.
-
MESSAGE_SENT
-
Constants for use with the displayEvent method.
-
MESSAGE_UNSENT
-
Constants for use with the displayEvent method.
-
REAL_TIME
-
Constants for use with the setDelay method.
-
SLOW
-
Constants for use with the setDelay method.
-
STATUS_CHANGE
-
Constants for use with the displayEvent method.
-
STEP_BY_STEP
-
Constants for use with the setDisplayMode method.
-
TIME_STEP
-
Constants for use with the setDisplayMode method.
-
TOP
-
Constant used in output()

-
MonitorGUI()

-
actionPerformed(ActionEvent)
-
Receives ActionEvents from the buttons in the GUI and handles them
-
adjustmentValueChanged(AdjustmentEvent)
-
Recieves AdjustmentEvents related to the slider used to step between visualisation
steps.
-
crossOutMessage(String,
String, Message)
-
Used to indicate that some failure has ocurred when delivering a Message.
-
deRegister(String)
-
Make the monitorable object with this name unmonitorable
-
displayEvent(int,
String, String, Message)
-
Informs the monitor of an 'event' to be displayed.
-
displayMessage(String,
String, Message)
-
Display a copy of a Message sent by a monitorable object.
-
getMessages()
-
The the set of Messages currently being shown by the Monitor
-
informOfFailure(String,
String, Message)
-
informOfMessage(String,
String, Message)
-
informOfStatus(String,
String)
-
init()
-
Initialise the GUI, the MonitorCanvas
-
itemStateChanged(ItemEvent)
-
Recieves ItemEvents from the choice boxes in the GUI, and handles them
accordingly
-
output(int,
String)
-
Output the string in one of the text areas shown by this GUI
-
output(String)
-
Output the string to the top text area shown by this GUI
-
register(Monitorable)
-
Registers a monitorable object that wishes to be monitored
-
setDelay(long)
-
Set the time delay for use in TIME_STEP mode.
-
setDisplayMode(int)
-
Set whether the GUI should show each visualisation step and wait for the
user to click 'next' - or whether it should run through the steps according
to a particular delay
-
statusChange(String,
String)
-
Indicates a change in status of a registered Monitorable object.
-
stop()
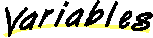
MESSAGE_SENT
public static final int MESSAGE_SENT
-
Constants for use with the displayEvent method.
-
See Also:
-
displayEvent
MESSAGE_UNSENT
public static final int MESSAGE_UNSENT
-
Constants for use with the displayEvent method.
-
See Also:
-
displayEvent
STATUS_CHANGE
public static final int STATUS_CHANGE
-
Constants for use with the displayEvent method.
-
See Also:
-
displayEvent
STEP_BY_STEP
public static final int STEP_BY_STEP
-
Constants for use with the setDisplayMode method.
-
See Also:
-
setDisplayMode
TIME_STEP
public static final int TIME_STEP
-
Constants for use with the setDisplayMode method.
-
See Also:
-
setDisplayMode
SLOW
public static final long SLOW
-
Constants for use with the setDelay method.
-
See Also:
-
setDelay
MEDIUM
public static final long MEDIUM
-
Constants for use with the setDelay method.
-
See Also:
-
setDelay
FAST
public static final long FAST
-
Constants for use with the setDelay method.
-
See Also:
-
setDelay
REAL_TIME
public static final long REAL_TIME
-
Constants for use with the setDelay method.
-
See Also:
-
setDelay
canvas
protected MonitorCanvas canvas
TOP
public static final int TOP
-
Constant used in output()
-
See Also:
-
output
BOTTOM
public static final int BOTTOM
-
Constant used in output()
-
See Also:
-
output

MonitorGUI
public MonitorGUI()
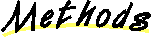
init
public void init()
-
Initialise the GUI, the MonitorCanvas
stop
public void stop()
getMessages
public String[] getMessages()
-
The the set of Messages currently being shown by the Monitor
output
public void output(String string)
-
Output the string to the top text area shown by this GUI
output
public void output(int where,
String string)
-
Output the string in one of the text areas shown by this GUI
-
Parameters:
-
where - either TOP or BOTTOM
setDisplayMode
public void setDisplayMode(int mode)
-
Set whether the GUI should show each visualisation step and wait for the
user to click 'next' - or whether it should run through the steps according
to a particular delay
-
See Also:
-
STEP_BY_STEP, TIME_STEP
setDelay
public void setDelay(long millis)
-
Set the time delay for use in TIME_STEP mode.
-
See Also:
-
SLOW, MEDIUM, FAST,
REAL_TIME
adjustmentValueChanged
public void adjustmentValueChanged(AdjustmentEvent evt)
-
Recieves AdjustmentEvents related to the slider used to step between visualisation
steps. Handles the events correctly.
actionPerformed
public void actionPerformed(ActionEvent e)
-
Receives ActionEvents from the buttons in the GUI and handles them
itemStateChanged
public void itemStateChanged(ItemEvent evt)
-
Recieves ItemEvents from the choice boxes in the GUI, and handles them
accordingly
displayEvent
public synchronized void displayEvent(int event_type,
String from,
String otherStr,
Message msg)
-
Informs the monitor of an 'event' to be displayed. This is synchronized
to enable unique time-stamping of these events, so as to ensure consistency
when tracing back/forwards through the currently stored events. The type
of event specified in event_type dictates the content of the other
parameters. The appropriate method is called to display the various types
of events
Depending on the current display mode and time delay in the Monitor,
this method will only return when this 'event' has been displayed. This
is to ensure that what is seen on the display is what is currently occuring
in the agent scenario -- as agents are 'blocked' until the event they wish
to visualise has has been displayed.
-
Parameters:
-
event_type - The type of event, valid values are MESSAGE_SENT, MESSAGE_UNSENT,
STATUS_CHANGE .
-
from - The name of the Monitorable object that this event concerns
-
otherStr - In the case of STATUS_CHANGE, this is the latest comment
string sent by 'from'. In the other cases, this is the recipient of the
Message specified in 'msg'.
-
msg - In the case of STATUS_CHANGE, this is ignored. In the other
cases, this is the Message object passed from 'from' to 'otherStr'
-
See Also:
-
MESSAGE_SENT, MESSAGE_UNSENT, STATUS_CHANGE, displayMessage, crossOutMessage,
statusChange
statusChange
protected void statusChange(String name,
String comment)
-
Indicates a change in status of a registered Monitorable object. Notifies
the MonitorCanvas of the change.
-
Parameters:
-
name - The name of the Monitorable object the change affects.
-
comment - The detail of the status change
-
See Also:
-
statusChange
crossOutMessage
protected void crossOutMessage(String from,
String to,
Message msg)
-
Used to indicate that some failure has ocurred when delivering a Message.
Notifies the MonitorCanvas of this. A Message that has previously been
displayed did not reach its destination, the effect of calling this will
be to 'cross out' the graphical representation of the message if it is
displayed on the MonitorCanvas again.
-
See Also:
-
crossOutMessage
displayMessage
protected void displayMessage(String from,
String to,
Message msg)
-
Display a copy of a Message sent by a monitorable object. The names 'from'
and 'to' are checked against registered Monitorables and the 'event' is
queued for displaying. The MonitorCanvas is notified of this Message, and
displays it according to the current display mode and time delay. To ensure
consistency with timing and race conditions when displaying different events,
this method is protected. Use displayEvent(MonitorGUI.MESSAGE_SENT,
from, to, msg) from non subclasses of this class.
-
See Also:
-
displayEvent, MESSAGE_SENT,
setDisplayMode, setDelay
register
public void register(Monitorable agent) throws RemoteException
-
Registers a monitorable object that wishes to be monitored
-
See Also:
-
MonitorServer
deRegister
public void deRegister(String name)
-
Make the monitorable object with this name unmonitorable
-
See Also:
-
MonitorServer
informOfMessage
public void informOfMessage(String from,
String to,
Message m)
-
See Also:
-
MonitorServer
informOfFailure
public void informOfFailure(String from,
String to,
Message m)
-
See Also:
-
MonitorServer
informOfStatus
public void informOfStatus(String name,
String comment)
-
See Also:
-
MonitorServer
All Packages Class Hierarchy This Package Previous Next Index